[dayjs] dayjs를 이용하여 Date 객체 잘 다루기
프로그래밍을 하면서 날짜 관련 작업을 하는 경우가 많이 있습니다.
간단한 경우는 Date 객체를 사용하면 되지만 복잡한 경우 날짜 관련 라이브러리를 사용하는 것이 간편합니다.
기존에는 moment를 사용하였지만 용량이 너무 크고 지원 중단이 되었습니다.
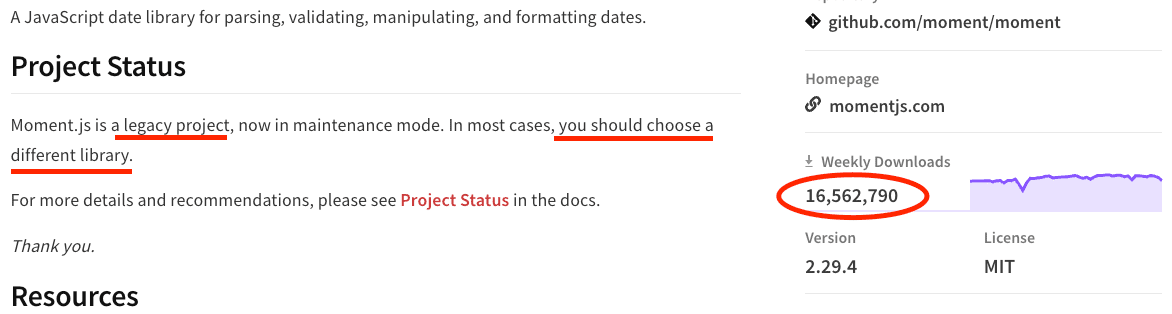
여러 대체 라이브러리를 찾다가 dayjs를 선택하였습니다.
dayjs의 특징은 extend를 이용하여 내가 원하는 플러그인을 추가하여 사용할 수 있다는 것입니다.
위 내용은 차차 알아보고 dayjs로 Date를 다루는 방법을 알아보겠습니다.
dayjs 설치
이 게시글을 보시는 분은 대부분 npm은 사용하실 줄 안다고 생각합니다.
따라서 설치 및 가져오기는 명령어만 작성하겠습니다.
◆ dayjs 설치하기
명령어 : yarn add dayjs or npm i dayjs
◆ dayjs 가져오기
명령어 : import dayjs from "dayjs" or const dayjs = require("dayjs")
dayjs 사용하기
dayjs()를 출력하면 아래 결과가 나옵니다.
const dayjs = require("dayjs");
console.log("dayjs : ", dayjs());
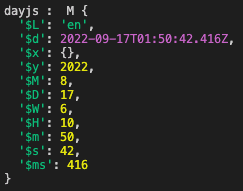
위 결과만보면 무슨 의미인지는 대충 알겠는데 어떻게 사용하지는 이해할 수 없습니다.
이제 이 dayjs로 Date 객체 가져오기, 원하는 Date 객체 넣어주기, date format 지정하기 등을 해보겠습니다.
◆ Date 객체 출력하기 & 설정하기
Date 객체를 생성하는 방법은 매우 간단합니다.
const dayjs = require("dayjs");
console.log("now : ", dayjs().toDate());

위 처럼 dayjs() 생성 시 추가적인 정보를 넘겨주지 않으면 현재 시간으로 생성됩니다.
만약 다른 시간 대의 dayjs 객체가 필요한 경우 인자에 넣어주시면 됩니다.
const dayjs = require("dayjs");
console.log("now : ", dayjs("2024-05-19T09:59:23.974Z").toDate());

위와 같이 특정 시간대의 Date를 넣어주면 해당 시간의 dayjs 객체가 생성됩니다.
◆ 년 월 일 시 분 초 출력하기
년, 월, 일, 시, 분, 초를 출력하는 방법은 간단합니다.
const dayjs = require("dayjs");
const now = dayjs();
const future = dayjs("2024-05-19T09:59:23.974Z");
console.log("------- 현재 시간 -------");
console.log(`${now.year()}년`);
console.log(`${now.month() + 1}월`);
console.log(`${now.date()}일`);
console.log(`${now.hour()}시`);
console.log(`${now.minute()}분`);
console.log(`${now.second()}초`);
console.log();
console.log("------- 미래 시간 -------");
console.log(`${future.year()}년`);
console.log(`${future.month() + 1}월`);
console.log(`${future.date()}일`);
console.log(`${future.hour()}시`);
console.log(`${future.minute()}분`);
console.log(`${future.second()}초`);
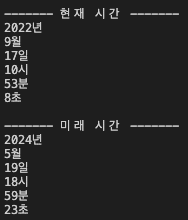
위 코드를 보면 어렵지 않게 년, 월, 일, 시, 분, 초를 출력할 수 있습니다.
그런데 특이한 점이 있습니다.
월(month)의 경우 0부터 시작하기 떄문에 +1 을 해주어야 합니다.
위 내용만 주의하시면 쉽게 사용할 수 있을 것입니다.
◆ 년 월 일 시 분 초 변경하기
년, 월, 일, 시, 분, 초를 변경하는 경우가 있을 수 있겠죠?
변경하는 방법도 간단합니다.
const dayjs = require("dayjs");
const now = dayjs();
console.log();
console.log(`변경 전 ${now.year()}년`);
console.log(`변경 후 ${now.set("year", 2002).year()}년\n`);
console.log(`변경 전 ${now.month() + 1}월`);
console.log(`변경 후 ${now.set("month", 7).month() + 1}월\n`);
console.log(`변경 전 ${now.date()}일`);
console.log(`변경 후 ${now.set("date", 17).date()}일\n`);
console.log(`변경 전 ${now.hour()}시`);
console.log(`변경 후 ${now.set("hour", 3).hour()}시\n`);
console.log(`변경 전 ${now.minute()}분`);
console.log(`변경 후 ${now.set("minute", 17).minute()}분\n`);
console.log(`변경 전 ${now.second()}초`);
console.log(`변경 후 ${now.set("second", 7).second()}초\n`);

위 코드에서 확인할 수 있듯이 set 함수를 이용하면 쉽게 변경할 수 있습니다.
◆ 년 월 일 시 분 초 내가 원하는 포맷으로 출력하기
위에서 년 월 일 시 분 초를 출력하는 방법을 확인해보았습니다.
그러나 위와 같이 출력하면 완성된 출력 결과를 만들기 힘듭니다.
이러한 경우 format 함수를 이용하면 쉽게 출력할 수 있습니다.
const dayjs = require("dayjs");
const now = dayjs();
console.log("------- 현재 시간 -------");
console.log(`${now.format("YYYY MM DD hh mm ss")}`);
console.log(`${now.format("YYYY.MM.DD.hh.mm.ss")}`);
console.log(`${now.format("YYYY-MM-DD.hh.mm.ss")}`);
console.log(`${now.format("YYYY년 MM월 DD일 hh시 mm분 ss초")}`);
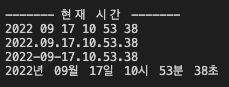
위와 같이 format 함수를 이용하여 출력하면 아주 쉽게 원하는 포맷으로 출력할 수 있습니다.
그러나 위 코드의 경우 시간이 제대로 출력되지 않을 수 있습니다.
예를들면 19시인 경우 19시가 아니라 07시로 표시가 됩니다.
이것을 수정하는 방법은 아주 간단합니다.
const dayjs = require("dayjs");
const now = dayjs();
console.log("------- 현재 시간 -------");
console.log(`${now.format("YYYY MM DD HH mm ss")}`);
console.log(`${now.format("YYYY.MM.DD.HH.mm.ss")}`);
console.log(`${now.format("YYYY-MM-DD.HH.mm.ss")}`);
console.log(`${now.format("YYYY년 MM월 DD일 HH시 mm분 ss초")}`);
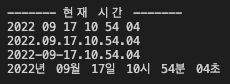
위 코드에서 확인할 수 있듯이 시간을 표시할 때 hh가 아니라 HH로 표시하면 됩니다.
그렇다면 AM PM 으로 오전 오후를 출력하고 싶으면 어떻게 할까요?
아래 코드를 확인해봅시다.
const dayjs = require("dayjs");
const now = dayjs();
console.log("------- 현재 시간 -------");
console.log(`${now.format("HH.mm a")}`);
console.log(`${now.format("HH.mm A")}`);
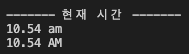
위 코드에서 확인할 수 있듯이 A를 사용하면 됩니다.
대소문자 구분도 가능합니다.
◆ 특정 기준으로 시작, 끝 설정하기
소제목 이름이 조금 이상한데 코드를 보시면 이해하실 수 있을 것입니다.
const dayjs = require("dayjs");
const now = dayjs();
console.log();
console.log("------- 현재 시간 -------");
console.log(`${now.format("YYYYMMDD HHmmss")}`);
console.log("\n------- day 기준 -------");
console.log(`${now.startOf("day").format("YYYYMMDD HHmmss")}`);
console.log(`${now.endOf("day").format("YYYYMMDD HHmmss")}`);
console.log("\n------- week 기준 -------");
console.log(`${now.startOf("week").format("YYYYMMDD HHmmss")}`);
console.log(`${now.endOf("week").format("YYYYMMDD HHmmss")}`);
console.log("\n------- year 기준 -------");
console.log(`${now.startOf("year").format("YYYYMMDD HHmmss")}`);
console.log(`${now.endOf("year").format("YYYYMMDD HHmmss")}`);
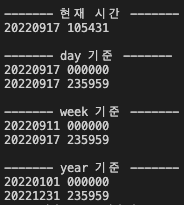
추가적인 설명은 하지 않겠습니다.
위와 같은 경우는 특정 기간 사이의 데이터가 있는 지 없는 지 확인할 때 사용합니다.
◆ 특정 Date 더하기 / 빼기
특정 날짜를 기준으로 원하는 시간만큼 더하거나 빼야하는 경우가 있습니다.
이러한 경우 add, subtract 함수를 사용하면 됩니다.
아래 코드를 확인해봅시다.
const dayjs = require("dayjs");
const now = dayjs();
console.log("------- 현재 / 1시간 더하기 / 빼기 -------");
console.log(`${now.format("YYYYMMDD HHmmss")}`);
console.log(`${now.add(1, "hour").format("YYYYMMDD HHmmss")}`);
console.log(`${now.subtract(1, "hour").format("YYYYMMDD HHmmss")}`);
console.log("\n------- 현재 / 10분 더하기 / 빼기 -------");
console.log(`${now.format("YYYYMMDD HHmmss")}`);
console.log(`${now.add(1, "minute").format("YYYYMMDD HHmmss")}`);
console.log(`${now.subtract(1, "minute").format("YYYYMMDD HHmmss")}`);
console.log("\n------- 현재 / 15초 더하기 / 빼기 -------");
console.log(`${now.format("YYYYMMDD HHmmss")}`);
console.log(`${now.add(1, "second").format("YYYYMMDD HHmmss")}`);
console.log(`${now.subtract(1, "second").format("YYYYMMDD HHmmss")}`);

위 코드에는 없지만 년, 월, 일도 당연히 가능합니다.
◆ Date 비교하기
Date 객체가 같은지? 이전인지? 이후인지? 확인해야하는 경우가 있습니다.
Date 비교 시 같거나 이전 or 같거나 이후인지를 확인하기 위해서는 플러그인을 추가해주어야 합니다.
아래 코드를 확인하면 extend로 isSameOrBefore, isSameOrAfter를 추가한 것을 확인할 수 있을 것입니다.
(같음, 이전, 이후만 비교하는 경우 필요 없음)
현재 시간과 현재 시간에 10시간, 10초를 더한 시간을 비교한 결과입니다.
const dayjs = require("dayjs");
const isSameOrBefore = require("dayjs/plugin/isSameOrBefore");
const isSameOrAfter = require("dayjs/plugin/isSameOrAfter");
dayjs.extend(isSameOrBefore);
dayjs.extend(isSameOrAfter);
const now = dayjs();
const future = now.add(10, "hour").add(10, "second");
console.log("------- 원본 -------");
console.log(now.format("YYYY MM DD HH mm ss"));
console.log();
console.log("------- 수정 -------");
console.log(future.format("YYYY MM DD HH mm ss"));
console.log();
console.log("------- 같은가? -------");
console.log("전체 비교", now.isSame(future));
console.log("년도 비교", now.isSame(future, "year"));
console.log("월 비교", now.isSame(future, "month"));
console.log("시 비교", now.isSame(future, "hour"));
console.log("초 비교", now.isSame(future, "second"));
console.log();
console.log("------- 이전인가? -------");
console.log("전체 비교", now.isBefore(future));
console.log("년도 비교", now.isBefore(future, "year"));
console.log("월 비교", now.isBefore(future, "month"));
console.log("시 비교", now.isBefore(future, "hour"));
console.log("초 비교", now.isBefore(future, "second"));
console.log();
console.log("------- 이후인가? -------");
console.log("전체 비교", now.isAfter(future));
console.log("년도 비교", now.isAfter(future, "year"));
console.log("월 비교", now.isAfter(future, "month"));
console.log("시 비교", now.isAfter(future, "hour"));
console.log("초 비교", now.isAfter(future, "second"));
console.log();
console.log("------- 같거나 이전인가? -------");
console.log("전체 비교", now.isSameOrBefore(future));
console.log("년도 비교", now.isSameOrBefore(future, "year"));
console.log("월 비교", now.isSameOrBefore(future, "month"));
console.log("시 비교", now.isSameOrBefore(future, "hour"));
console.log("초 비교", now.isSameOrBefore(future, "second"));
console.log();
console.log("------- 같거나 이후인가? -------");
console.log("전체 비교", now.isSameOrAfter(future));
console.log("년도 비교", now.isSameOrAfter(future, "year"));
console.log("월 비교", now.isSameOrAfter(future, "month"));
console.log("시 비교", now.isSameOrAfter(future, "hour"));
console.log("초 비교", now.isSameOrAfter(future, "second"));
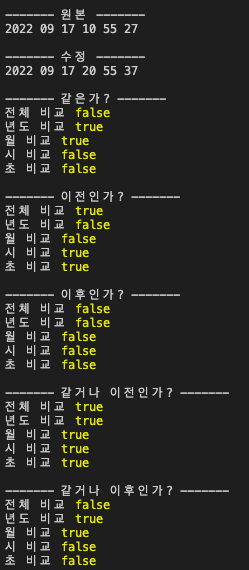
코드에서 볼 수 있듯이 시간을 비교할 때 비교할 기준을 정할 수 있습니다.
적절히 필요한 기준을 넘겨주면 됩니다.
◆ Date 차이 구하기
특정 시간끼리의 차이를 구해야하는 경우가 있습니다.
이러한 경우 diff 함수를 사용하면 쉽게 구할 수 있습니다.
const dayjs = require("dayjs");
const now = dayjs();
const future = now
.add(1, "year")
.add(1, "month")
.add(10, "hour")
.add(10, "second");
console.log("millisecond", future.diff(now));
console.log("year", future.diff(now, "year"));
console.log("month", future.diff(now, "month"));
console.log("day", future.diff(now, "day"));
console.log("hour", future.diff(now, "hour"));
console.log("minute", future.diff(now, "minute"));
console.log("second", future.diff(now, "second"));
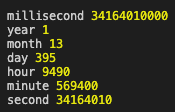
◆ 윤년 확인하기
컴퓨터 공학과인 경우 학창시절 윤년 구하기 많이 해보셨을 것입니다.
윤년도 isLeapYear 함수를 이용하면 쉽게 구할 수 있습니다.
isLeapYear 함수를 사용하기 위해서는 extend를 해주어야 합니다.
(아래 코드에서 확인)
const dayjs = require("dayjs");
const isLeapYear = require("dayjs/plugin/isLeapYear");
dayjs.extend(isLeapYear);
console.log("2000년 : ", dayjs("2000-01-01").isLeapYear());
console.log("2001년 : ", dayjs("2001-01-01").isLeapYear());
console.log("2002년 : ", dayjs("2002-01-01").isLeapYear());
console.log("2003년 : ", dayjs("2003-01-01").isLeapYear());
console.log("2004년 : ", dayjs("2004-01-01").isLeapYear());
console.log("2005년 : ", dayjs("2005-01-01").isLeapYear());
console.log("2006년 : ", dayjs("2006-01-01").isLeapYear());
console.log("2007년 : ", dayjs("2007-01-01").isLeapYear());
console.log("2008년 : ", dayjs("2008-01-01").isLeapYear());
console.log("2009년 : ", dayjs("2009-01-01").isLeapYear());
console.log("2010년 : ", dayjs("2010-01-01").isLeapYear());
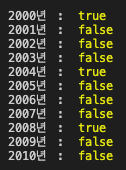
◆ 1년 중 몇 번째 week 인지 구하기
week를 구하는 방법은 2가지 있습니다.
weekOfYear, isoWeek 플러그인을 추가해줍니다.
week의 경우 해당 년도에서 몇 번째 week인지 계산
isoWeek의 경우 iso 표준에 맞추어 week를 계산합니다.
아래 코드 결과를 보면 이해하실 수 있으실 것입니다.
const dayjs = require("dayjs");
const weekOfYear = require("dayjs/plugin/weekOfYear");
const isoWeek = require("dayjs/plugin/isoWeek");
dayjs.extend(weekOfYear);
dayjs.extend(isoWeek);
const date_2022_12_31 = dayjs("2022-12-31");
const date_2023_01_01 = dayjs("2023-01-01");
const date_2023_12_31 = dayjs("2023-12-31");
const date_2024_01_01 = dayjs("2024-01-01");
console.log(`2022_12_31 : ${date_2022_12_31.week()}`);
console.log(`2023_01_01 : ${date_2023_01_01.week()}`);
console.log(`2022_12_31 : ${date_2022_12_31.isoWeek()}`);
console.log(`2023_01_01 : ${date_2023_01_01.isoWeek()}`);
console.log();
console.log(`2023_12_31 : ${date_2023_12_31.week()}`);
console.log(`2024_01_01 : ${date_2024_01_01.week()}`);
console.log(`2023_12_31 : ${date_2023_12_31.isoWeek()}`);
console.log(`2024_01_01 : ${date_2024_01_01.isoWeek()}`);
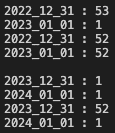
끝
위 정도 개념만 알면 dayjs로 Date를 다룰 때 큰 어려움이 없으실 것입니다.
만약 막히거나 궁금한 점이 있으면 공식 문서를 확인하시면 됩니다.
참고자료
마지막
해당 내용은 틀릴 수도 있습니다. 틀린 내용이 있으면 조언 부탁드립니다.
'공유 > JavaScript, TypeScript' 카테고리의 다른 글
[TypeScript] 타입스크립트 union 타입 합치기 (0) | 2024.01.16 |
---|---|
[JavaScript] Binary 데이터 이미지로 변환하기 (0) | 2022.09.23 |
[JavaScript] 문자열 반복문 돌리기, 문자열을 배열로 변환하기 (2) | 2022.04.04 |
[JavaScript] 배열 원소 swap, 요소 swap (0) | 2022.03.23 |
[JavaScript] async await로 비동기 처리 개선하기 (0) | 2022.03.02 |